To retrieve the latest version of your app from the Google Play Store using the Google Play Developer API
To retrieve the latest version of your app from the Google Play Store using the Google Play Developer API, you will need to follow these steps:
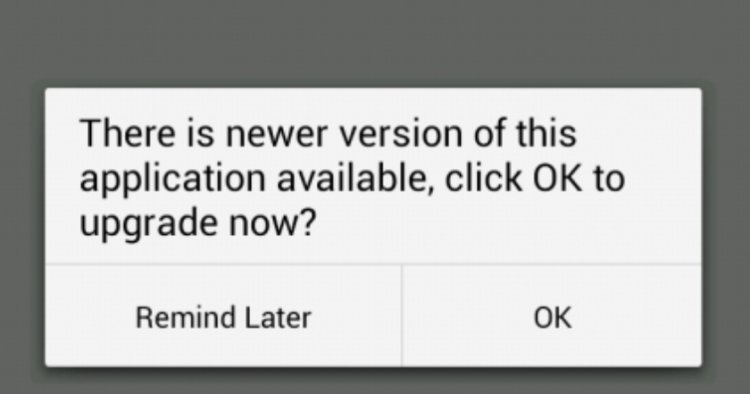
- Set up Google Play Developer API:
Go to the Google Play Console.
Create a new project or select an existing project.
Enable the Google Play Developer API for your project by following the instructions provided in the API documentation. - Obtain the necessary credentials:
Create credentials for your project in the Google Cloud Console.
Choose the appropriate credential type (e.g., service account) and follow the instructions to create and download the JSON key file containing the necessary credentials. - Add dependencies to your Android project:
In your app's build.gradle file, add the following dependencies:
dependencies { implementation 'com.google.api-client:google-api-client:1.31.5' implementation 'com.google.oauth-client:google-oauth-client-jetty:1.31.5' }
Write code to retrieve the latest version using the Google Play Developer API:
Place the downloaded JSON key file in your Android project's app folder. - Use the following code snippet to retrieve the latest version:
Place the downloaded JSON key file in your Android project's app folder.
Use the following code snippet to retrieve the latest version:
import com.google.api.client.googleapis.auth.oauth2.GoogleCredential; import com.google.api.client.googleapis.javanet.GoogleNetHttpTransport; import com.google.api.client.http.HttpRequestInitializer; import com.google.api.client.http.javanet.NetHttpTransport; import com.google.api.services.androidpublisher.AndroidPublisher; import com.google.api.services.androidpublisher.AndroidPublisherScopes; import com.google.api.services.androidpublisher.model.AppEdit; import com.google.api.services.androidpublisher.model.Track; import java.io.File; import java.io.IOException; import java.security.GeneralSecurityException; import java.util.Collections; // ... private String getLatestVersionFromPlayStore() { try { // Load credentials from the JSON key file GoogleCredential credential = GoogleCredential .fromStream(new FileInputStream(new File("path_to_json_key_file.json"))) .createScoped(Collections.singleton(AndroidPublisherScopes.ANDROIDPUBLISHER)); // Initialize the AndroidPublisher service NetHttpTransport httpTransport = GoogleNetHttpTransport.newTrustedTransport(); HttpRequestInitializer requestInitializer = credential; AndroidPublisher publisher = new AndroidPublisher.Builder(httpTransport, JSON_FACTORY, requestInitializer) .setApplicationName("YourAppName") .build(); // Retrieve the current app edit AndroidPublisher.Edits edits = publisher.edits(); AndroidPublisher.Edits.Insert insertRequest = edits.insert("your_package_name", null); AppEdit appEdit = insertRequest.execute(); // Retrieve the track information AndroidPublisher.Edits.Tracks tracks = edits.tracks(); Track track = tracks.get("your_package_name", appEdit.getId(), "your_track_name").execute(); // Get the latest version from the track String latestVersion = track.getReleases().get(0).getVersionCodes().get(0); // Commit and close the app edit edits.commit("your_package_name", appEdit.getId()).execute(); return latestVersion; } catch (IOException | GeneralSecurityException e) { e.printStackTrace(); } return null; }
Replace "path_to_json_key_file.json" with the actual path to the JSON key file you downloaded.
Replace "your_package_name" with the package name of your app.
Replace "your_track_name" with the desired track name (e.g., "alpha", "beta", "production")