Processing One Billion Rows in PHP
Processing one billion rows of data in PHP can be a challenging task due to memory limitations and performance concerns. However, it's possible with careful optimization and the right techniques. Here's a general approach you can take
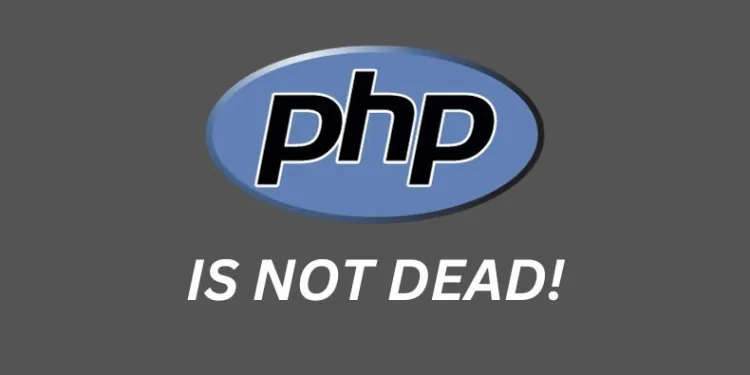
Processing one billion rows of data in PHP can be a challenging task due to memory limitations and performance concerns. However, it's possible with careful optimization and the right techniques. Here's a general approach you can take:
-
Use a Database: PHP itself may not be the best tool for handling such a large amount of data. Utilize a database like MySQL, PostgreSQL, or MongoDB, which are designed to efficiently handle large datasets.
-
Optimize Queries: Write optimized SQL queries that retrieve only the necessary data. Use indexes appropriately to speed up queries.
-
Batch Processing: Instead of trying to process all one billion rows at once, break the task into smaller batches. Retrieve, process, and store a manageable chunk of data at a time.
-
Streaming: If your database supports it, use streaming methods to fetch data from the database instead of loading it all into memory at once. This can significantly reduce memory usage.
-
Memory Management: If you need to process data in memory, be mindful of memory usage. Unset variables and objects when they are no longer needed to free up memory.
-
Parallel Processing: If possible, parallelize the processing task to take advantage of multiple CPU cores. You can achieve this using PHP extensions like pthreads or by using external tools like Gearman.
-
Optimize Code: Write efficient and optimized PHP code. Avoid unnecessary loops, function calls, and memory-intensive operations.
-
Caching: Use caching mechanisms like Redis or Memcached to store intermediate results and reduce the load on the database.
-
Profiling and Monitoring: Profile your code to identify bottlenecks and optimize performance. Monitor memory usage, CPU usage, and disk I/O to ensure efficient processing.
-
Consider Alternative Technologies: Depending on the nature of your task, PHP might not be the best tool for the job. Consider using languages or frameworks better suited for data processing, such as Python with Pandas or Apache Spark.
Here's a basic example of how you might process data in batches using PHP and MySQL:
<?php
$batchSize = 1000;
$offset = 0;
// Connect to the database
$pdo = new PDO('mysql:host=localhost;dbname=mydatabase', 'username', 'password');
// Process data in batches
while (true) {
$stmt = $pdo->prepare("SELECT * FROM mytable LIMIT $offset, $batchSize");
$stmt->execute();
$rows = $stmt->fetchAll(PDO::FETCH_ASSOC);
if (empty($rows)) {
break; // No more rows to process
}
foreach ($rows as $row) {
// Process each row
// Your processing logic goes here
}
$offset += $batchSize;
}
?>
Remember to adjust the batch size according to your server's memory constraints and processing capabilities.